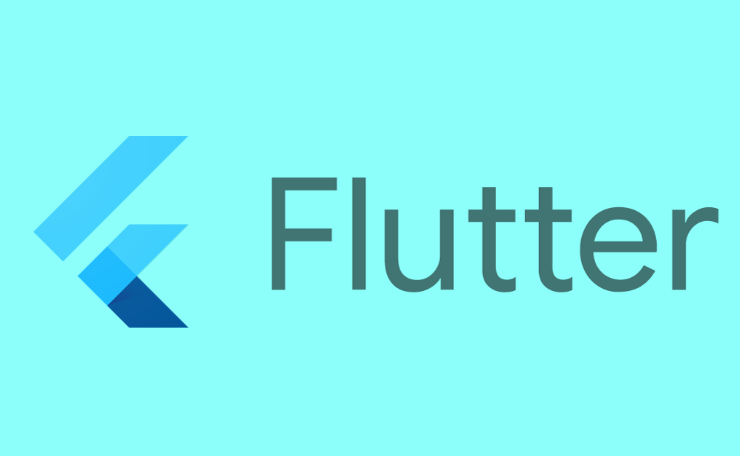
Flutter App Development (Beginner and Intermediate)
- Certificate upon completion
- 5 lessons
- 3 Days
Course Overview
Flutter, leveraging the Dart programming language, empowers developers to craft exceptional cross platform applications. This comprehensive course is designed for individuals who are already well-versed in Flutter development, with the goal of enhancing their expertise by exploring advanced architectural concepts and industry best practices.
Upon completing this course, participants will have honed the skills necessary to build resilient Flutter applications that can seamlessly scale to accommodate a substantial user base. Furthermore, they will acquire the knowledge to expand the reach of their applications beyond mobile devices, extending their capabilities to encompass web and desktop platforms.
Course Content
1. Introduction to Flutter
- Why Flutter Development
- Download Android Studio
- Windows Flutter Installation
2. Data Programming Language – Part 1
- Introduction
- Code style and naming convention
- Declaring variables
- Dart types and assigning types to variables
- Booleans
- The const and final keywords
- Concatenation
- Arithmetic Operators
- Relational Operators
3. Dart Programming Language – Part 2
- Control flow – If statements
- Logical Operators
- For Loops
- While, Do-While and Break
- Switch case
- Introduction to functions
- Function return types
- Using => operator for returning expressions
- Arguments and Functions
- Optional Parameters
- Lexical Scope
4. Object Oriented Programming
- Introduction to Classes and Objects
- Class creation and instance variables
- Adding methods
- Constructors, named and sugar syntactic constructors
- Setters and Getters
- Inheritance
- Override methods
- The toString() method
- Abstract and Interface classes
5. Data Structures and Collections
- Introduction to Collections
- Creating lists and iterating through them
- Creating list with a person type object
- Maps
6. Introduction to Flutter Widgets
- Introduction
- Flutter Hello World App
- Understanding material design
- Formatting code
- Material design presentation
- Widgets and properties
- Event listeners
- Custom controls
- Bottom navigator and a tab
- Floating action buttons
- Build a BMI Calculator
- Stateless Widgets
- Stateful Widgets
7. Flutter Multiple Page Concepts
- Creating a Listview
- Customizing ListTitle Row and adding a Tap
- Navigating to a second route
- Returning to the first route
- Passing data to the second route
- Passing data from the second route
- Model
- Passing objects
- Customizing Rows
- Adding Images
- Customizing colors
8. Understanding Themes
- Introduction to UI and UX
- Material Design
- Themes in Flutter
- Theming headlines and body text
- Customizing fonts
- Refactoring text theme
- Material design
- Material design – color themes
- Material design – Typography and Iconography
9. Local Persistence
- Flutter Local Persistence
- Local Storage
- SQLite
- Other persistence options: FIlestorage, Drift
10. Connecting to the World
- Introduction to HTTP and JSON
- Async and Future – Http Requests
- Adding Http Packages
- Setup network class
- Fetching JSON Data
- Fetching JSON using future builder widget
- Finalizing Fetching JSON
- Plain Old Dart Object (PODO)
- Mapping PODO with JSON
- Final Implementation
11. Firebase with Flutter
- Overview of Firebase services: Authentication, Firestore, Storage
- Setting up a Flutter project with Firebase
- Integrating Firebase Authentication
- Hands-on: Implementing Firebase Authentication in an app
- Understanding Firebase Cloud Firestore
- Reading and writing data to Firestore
- Utilizing StreamBuilder for real-time data updates
- Hands-on: Creating a real-time chat application with Firestore and StreamBuilder
12. State Management with Flutter
- Understanding the Provider package
- Setting up a Provider for state management
- Managing app-wide state using ChangeNotifier
- Hands-on: Creating a to-do list app with Provider
13. Publishing Flutter Apps
- Creating App Icon
- Dynamically generating icons using plugins
- Adding splash screen
- Adding launcher images
- Publishing App to Google Play Store
- Demonstration on App Publishing to App Store
Course Objective
- Develop iOS and Android applications proficiently
- Master Dart and the Flutter Framework
- Create complete and feature-rich mobile apps
- Build a portfolio of apps for job applications
- Become a cross-platform developer for iOS , Android and Desktop application
- Gain a competitive advantage as a Flutter mobile developer in the workplace
Testimonials
Testimonials

"If you get the chance to enter this bootcamp, consider yourself extremely lucky as they will groom you to have job ready skills. I have learnt a lot from this place."

“I learned many things when I was with this company. such as HTML, CSS, and JS.
The staff also very friendly and comfortable to ask anything i wanted.”

"While following the program for 3 days, I was able to learn a variety of knowledge, especially in the module involving the backend."

"The training is interesting because the way the trainer delivers is very good, easy to understand and casual. The staffs are also very friendly and kind. Many reference materials are shared for trainee reference. The training is hands-on based and two way communication, where very suitable for this course."

"The Moose Academy is highly recommended, should you be interested in learning mobile application development from scratch! Sir Wan Muzaffar is an excellent trainer as he tailor-made each training session to fit his clients’ requirements and understanding levels! On top of that, the training materials provided are informative and easily understandable. My heartiest gratitude to Sir Wan Muzaffar, for all the mobile app knowledge and tricks that he had shared with me, throughout the interactive training session!"